iOS API Reference
Since version 2013 Winter Release the Fabasoft app.telemetry SDK was extended to also support for mobile devices. In this case Apple iOS apps written in Objective-C can be instrumented using the Fabasoft app.telemetry SDK.
This SDK for iOS is technically very similar to the Android Java SDK (see “Android API Reference”) except for the programming language which is Objective-C.
Prerequisites for Instrumenting iOS Apps
In order to use the Fabasoft app.telemetry SDK for mobile devices (iOS) you will need an installation of Fabasoft app.telemetry (Server, Agent + WebAPI) and ensure that the WebAPI web service is reachable from your mobile device by checking the WebAPI status page of your installation (https://<your server>/web.telemetry). This installation will receive the telemetry data and will be used to configure the infrastructure (agent, log pools, etc.).
Configuration for iOS Apps / XCode
The next required step is to include the Fabasoft app.telemetry SDK library for iOS (binary and header-files) as additional library into your project. All required library files are delivered with the app.telemetry installation media in the “Developer/iOS” folder.
Your project has to be configured the following way:
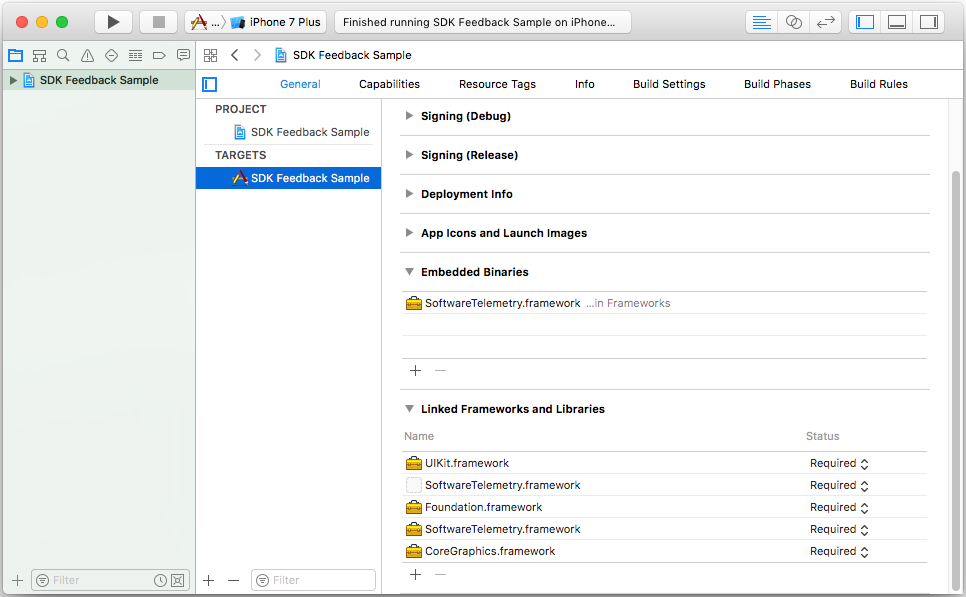
- Add the SoftwareTelemetry.framework-Framework to the Embedded Binaries and Linked Frameworks and Libraries of your targets general settings
- Import Software-Telemetry SDK main header file in your project source files:
- #import <SoftwareTelemetry/SoftwareTelemetry.h>
Instrumenting iOS Apps
The technical background for instrumenting any application is very similar for all languages so for example see chapter “”
As already described for the Android Java SDK (see chapter “Android API Reference”) the data transport is HTTP-based and requires a configuration URL to your WebAPI installation on initializing your API for your application. Also most of the methods are thread-based and require a thread-token to be passed as additional parameter.
In order to init the iOS SDK for your mobile app you need to pass the target URL of your app.telemetry WebAPI instance.
Syntax (Initialization) |
---|
NSString *serverUrl = @" https://<your server>/web.telemetry "; APM *apm = [APM initializeWithURL:serverUrl]; [apm registerApplicationWithName:@"Fabasoft app.telemetry" applicationId:@"iOS Sample" applicationTierName:@"Apple iOS SDK" applicationTierId:@"1"]; |
In the following code snippet is an extract from the sample project listing some of the main SDK functions of the iOS SDK.
Usage Example (MyViewController.m) |
---|
#import "MyViewController.h" #import <SoftwareTelemetry/SoftwareTelemetry.h> #import <QuartzCore/QuartzCore.h> // required for screenshot @implementation MyViewController // ... - (void)viewDidLoad { [super viewDidLoad]; //change the following URL to YOUR app.telemetry server/WebAPI //it is the URL where the telemetry data and the feedback is sent to NSString *serverUrl = @" https://<apptelemetry server>/web.telemetry"; APM *apm = [APM initializeWithURL:serverUrl]; [apm registerApplicationWithName:@"Fabasoft app.telemetry" applicationId:@"iOS Sample" applicationTierName:@"Apple iOS SDK" applicationTierId:@"1"]; APMModule *apmMod = [apm getModule:@"TestModule"]; [apmMod registerEventWithId:900 name:@"Event" parameters:@"text"]; [apmMod registerEventWithId:901 name:@"send Message" parameters:@"message"]; [apmMod registerEventWithId:910 name:@"Test Event" parameters:@""]; [apmMod registerEventWithId:912 name:@"Event with Params" parameters:@"p1;p2;p3"]; // ... [self fireSampleRequests:0]; } - (IBAction)fireSampleRequests:(id)sender { APM *apm = [APM instance]; APMModule *apmMod = [apm getModule:@"TestModule"]; // ... // request 1 uint64_t ctx = [apm createContextTx:0 filterValue:self.userName]; [apmMod eventTx:ctx eventId:900 level:APMLogLevelLog flags:APMFlagNone stringValue:@"My 1st Event"]; [apmMod eventTx:ctx eventId:901 level:APMLogLevelLog flags:APMFlagNone stringValue:greeting]; [apmMod eventTx:ctx eventId:920 level:APMLogLevelDetail flags:APMFlagEnter|APMFlagWait stringValue:@"50"]; usleep(50000); [apmMod eventTx:ctx eventId:920 level:APMLogLevelDetail flags:APMFlagLeave|APMFlagWait]; [apm releaseContextTx:ctx]; } - (IBAction)doReport:(id)sender { APM *apm = [APM instance]; APMModule *apmMod = [apm getModule:@"TestModule"]; uint64_t ctx = [apm createContextTx:0 filterValue:self.userName]; NSString *repk = [[NSString alloc] initWithFormat:@"REPK_%d", arc4random_uniform(10000)]; NSString *nameString = self.textField.text; NSString *descr = [[NSString alloc] initWithFormat:@"%@", nameString]; [apm reportTx:ctx filterValue:self.userName reportKey:repk description:descr]; [apm reportValueTx:ctx reportKey:repk valueKey:@"Rep_Val1" value:@"Report Value 1"];
if ([self.switchScreenshot isOn]) { [apmMod eventTx:ctx eventId:935 level:APMLogLevelDetail flags:APMFlagEnter|APMFlagWait]; APMReportContent *screenshot = [APM createScreenshotForView:self.view]; [apmMod eventTx:ctx eventId:935 level:APMLogLevelDetail flags:APMFlagLeave|APMFlagWait]; NSString *screenshotSize = [[NSString alloc] initWithFormat:@"%lu", (unsigned long)[[screenshot content] length]]; [apmMod eventTx:ctx eventId:931 level:APMLogLevelDetail flags:APMFlagNone stringValue:screenshotSize]; [apm reportContentWithKey:repk content:screenshot]; self.labelResult.text = [[NSString alloc] initWithFormat:@"Report sent with screenshot: '%@'", descr]; } else { [apmMod eventTx:ctx eventId:900 level:APMLogLevelDetail flags:APMFlagNone stringValue:@"Skipping Screenshot"]; self.labelResult.text = [[NSString alloc] initWithFormat:@"Report sent - NO screenshot: '%@'", descr]; } [apm releaseContextTx:ctx]; } |